Get Started
Chunkr AI
Open Source Document Intelligence
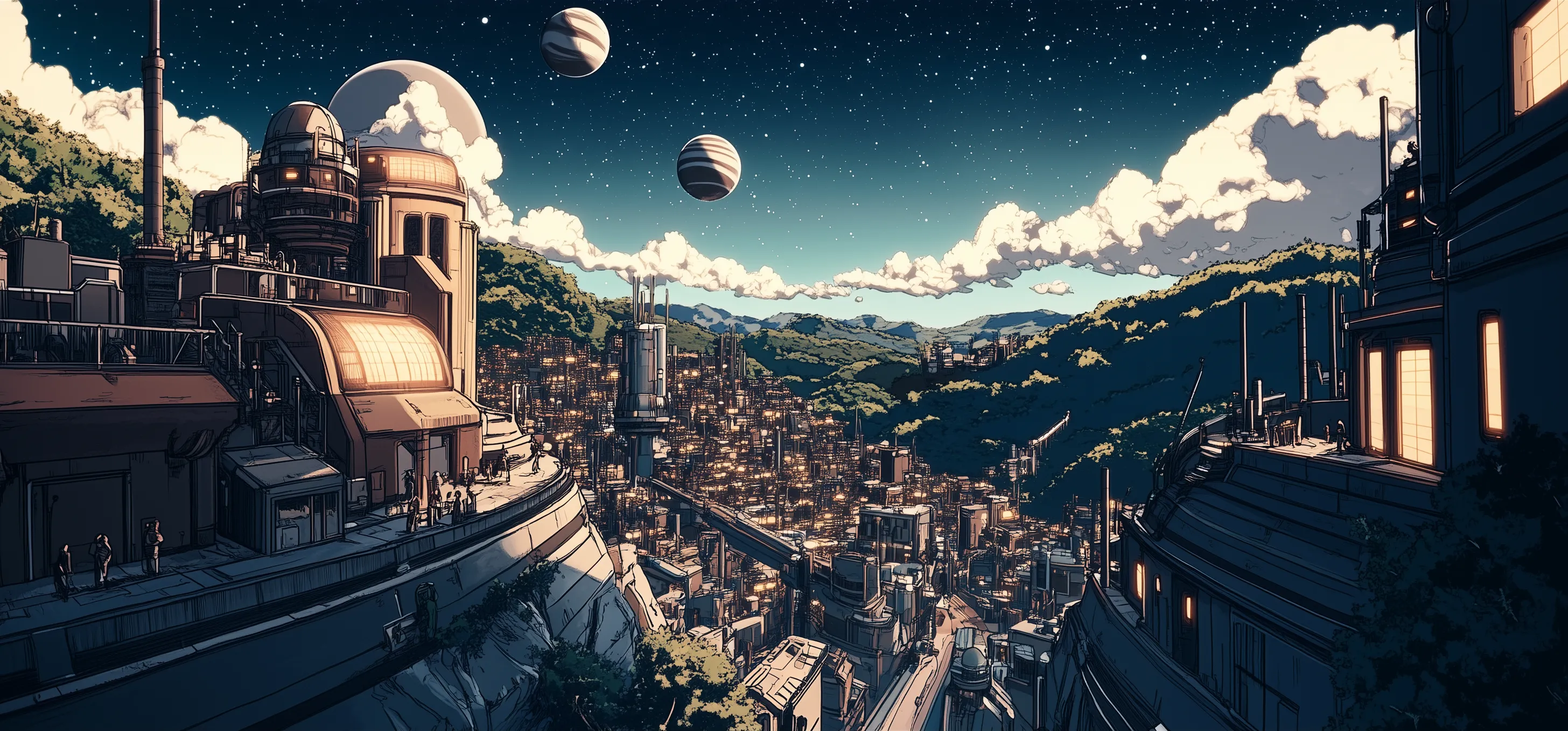
Quickstart
Learn how to get started with Chunkr AI API
Use Cases
Explore examples and strategies
SDKs
Access our client libraries
API Reference
Dive into our API reference
Features
Layout analysis
Preserve document structure with advanced layout detection
Segment processing
Leverage Vision Language Models for enhanced document understanding
OCR
Extract text from images and scanned documents with high accuracy
Intelligent chunking
Split documents into meaningful sections using layout-aware algorithms
Pipelines
Options for layout analysis and OCR providers
Multiple content types
Process PDFs, Office files (Word, Excel, PowerPoint), and images through a single API